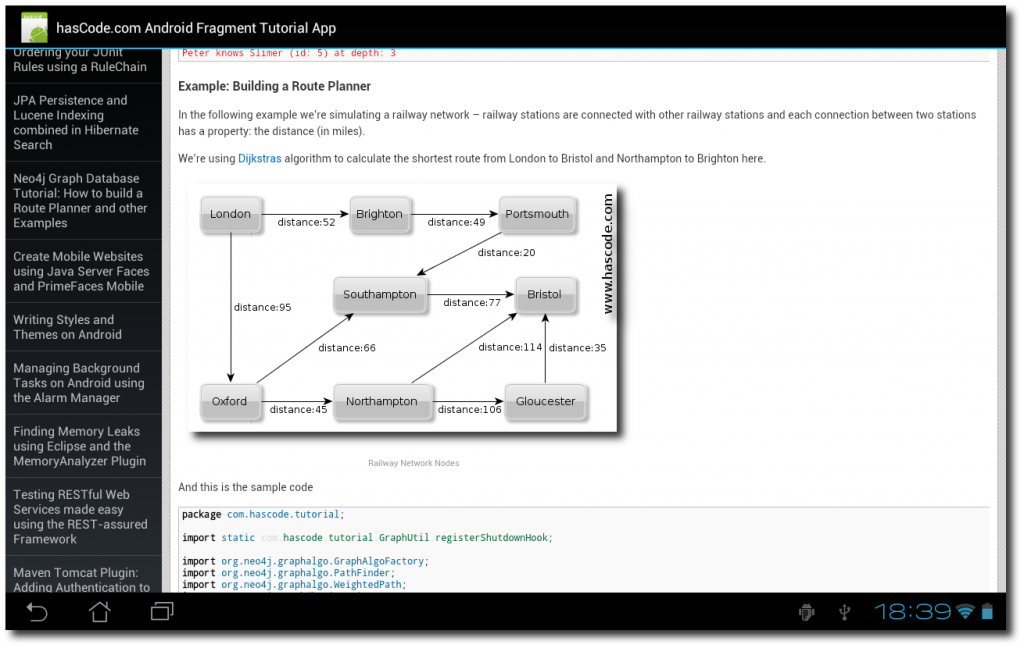
Using the Android Fragment API in a Tablet App
Since I got a new tablet running Android 4.0 aka ice cream sandwich I wanted to play around a bit with the fragments API and creating an application for a tablet. In the following tutorial, we’re going to build an application that renders several articles from a popular tech blog (just kidding) in a web view. What to build We’re going to build an application that is able to display web links in the left section of the screen and when clicked, displaying the corresponding website content in the right section of the application screen. ...