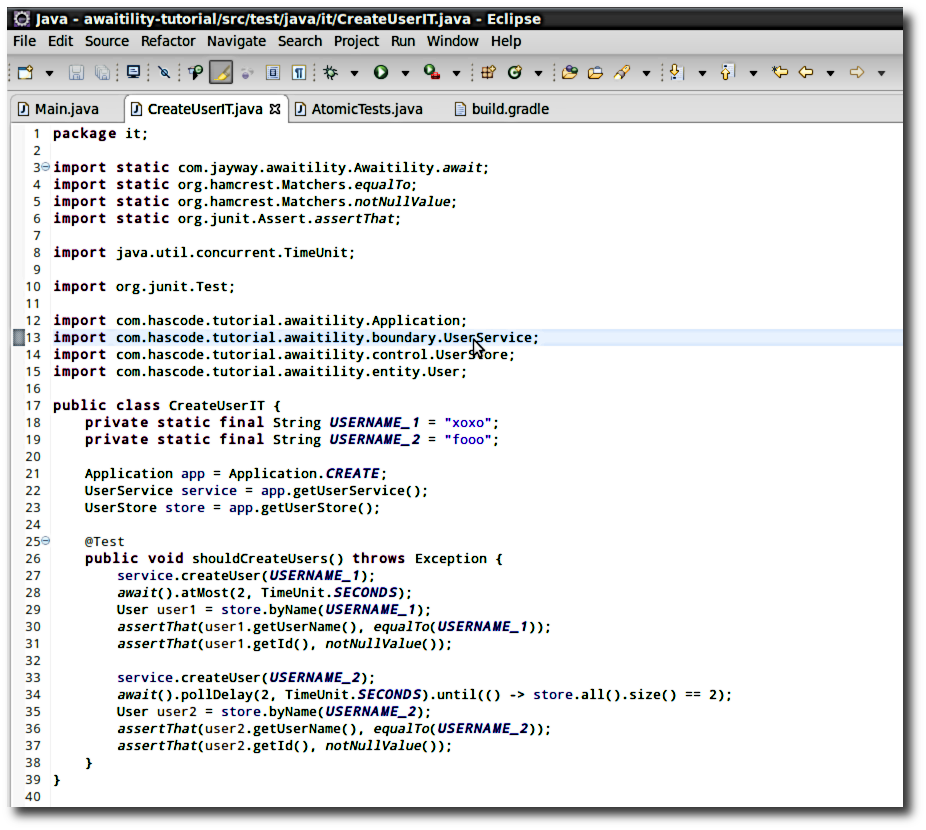
Testing Asynchronous Applications with Java and Awaitility
Writing tests for asynchronous applications has never been much fun as we’re always struggling with the problem how to determine state changes, handle process terminations, dealing with timeouts or failures and stuff like this. Awaitility eases this process for us offering a nice DSL, rich support for languages like Scala or Groovy and an easy-to-use syntax that’s even more fun when using it with Java 8′s lambda expressions. In the following short introduction I’d like to demonstrate writing some tests different scenarios. ...