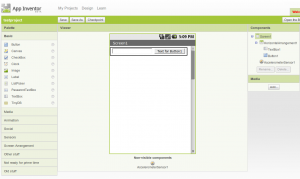
How to create an Android App using Google’s App Inventor
Today we’re going to take a look at Google’s App Inventor feature that offers programming-novices a nice possibility to enter the fabulous world of Android App programming without deeper knowledge of the API or complex SDK installations. So lets build some stuff .. Prerequisites Java 6 JDK App Inventors Extras Software A Google App Inventor Beta Account – request one here What we are going to build We are building a simple GUI with a Textbox and a button A click on the button starts an event that queries the acceleration sensor for coordinates If the sensor is active and enabled then the coordinates are displayed in the text box ...